Detect start of a loop in linked list
Problem :
Given a linked list, implement an algorithm which returns the node at the beginning of the loop.
This post is a follow-up of
- JavaScript Linked List Example
- Detect a loop in cyclic/circular linked list.
- Loop Length in cyclic/cicular linked list.
I recommend reading those posts first, as the following code uses the methods from it.
Logic:
- Find the loop, using the same logic Detect a loop in cyclic/circular linked list.
- Move
p1
at the head of the linked list, and keepp2
at the same location wherep1
andp2
met. - Move
p1
,p2
one step at a time, when they will meet again it's the beginning of the loop.

Watch the following video to understand the Floyd's cycle-finding algorithm!
This solution is a follow-up of JavaScript Linked List Example. I recommend reading it first, as the following code uses the method from it.
Solution :
Output :
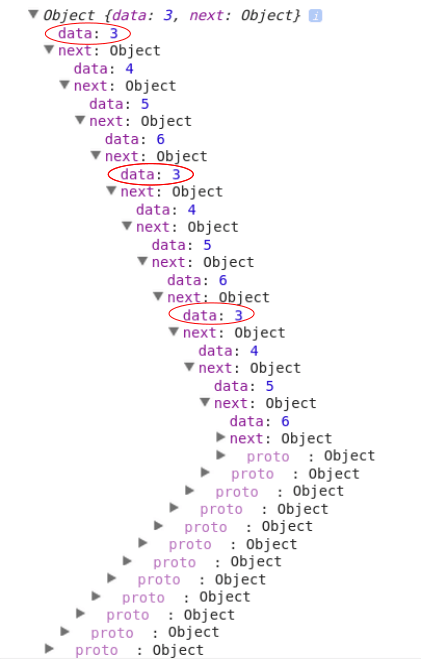